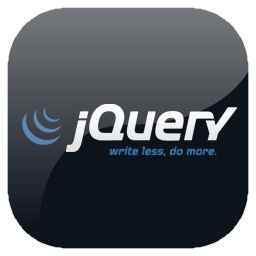
jQuery makes it super easy to get a reference to a collection of DOM elements. The jquery.each method allows you to execute a function against each one of the elements in the collection.
Solving problems is one of jQuery’s best qualities, and the beauty of the jQuery.each method is that it solves a very common problem: how to iterate a collection of DOM elements. Now in order to work through this task, one might be inclined to set up a for-loop. This approach is not at all wrong; in fact, on some levels it makes perfect sense. The thing is, in tackling it this way you run into a few avoidable problems. First, there is a lot of boilerplate code. In other words, to set up the for-loop you would need a counter variable, which we’ll call “i”. And since we might want to iterate a collection of DOM elements more than once, we’d need to make the “i” variable private, which means using a function to create private or “local” scope. Also, 80% of our for-loop would be repeated code. So, you get the picture… our problems are mounting, and they most assuredly are avoidable. So, here’s where the beauty of the jQuery.each method comes into play: it provides abstraction for creating a for-loop. This means that we need only provide the collection and a callback method that we wanted executed for each iteration of the loop.
The jQuery.each method is the go-to tool when you need to iterate a collection of DOM elements. Let’s say you have a bunch of elements in the page. Maybe that bunch is “every <a> tag” or “every <li> inside of the <ul> with the class sales”, or each <div> element that is a child of <div class=”members”>.
Here is how you would create a reference to each of those collections
jQuery will return a collection with one or more members. But how do you iterate over that collection and “do something” to each one? You can accomplish this by chaining the .each() method to the return value, and of course, pass a callback to that method.
jQuery.each Method – Basic Syntax
In the above example, where you see “selector”, that represents a CSS-like query such as “p” or “#some-id p img”, etc., that you would pass to jQuery. Now you can see that we have passed a callback function to the each() method. And inside of that callback, we would take care of any tasks that are specific to the elements over which we are currently iterating.
So, for an example, we will create a click-event handler for each <li> inside of a <ul>. Here is the markup we will work with:
With that markup in mind, consider the following code:
Example # 1
Notice that in Example # 1 we use $(this). Now just in case you are not familiar with this pattern, $(this) equals “the current element”, and it happens twice in our code. The first instance of $(this) on line # 2 refers to the element that is currently being iterated over (i.e. the current “UL LI”). The second $(this) on line # 3 represents the element that was just clicked. This is because we want to change the color of the element that was just clicked to red.
2 – In the click-event handler, we say “If you click me, make my text red”
Example # 2
In Example # 2, we change the logic a bit so that only an <li> with the class “workday” is returned in the collection, which means that the “saturday” and “sunday” <li> elements do have a click event handler assigned.
Here is the JsFiddle link for this example: http://jsfiddle.net/WHkkA/. Simply remove the .workday class from the CSS selector to see Example # 1 work.
Summary
The jQuery .each() method is used to iterate over the members of a jQuery collection. There is definitely more fun stuff that can be done with this method, but this is just a very basic overview of what the method does and how to use it.
Important Note: Try not to confuse this with the jQuery.each() method. That is a similar concept, but more of a general-purpose iteration method that is a static method call to the jQuery object, not a method of a jQuery collection.