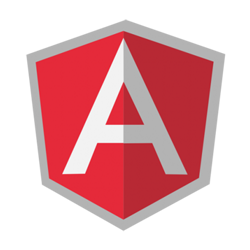
Data-binding with Angular.js is surprisingly simple. To get started, you need only specify an ng-model directive.
One of the most common tasks for any JavaScript developer is to inject a value into the DOM. This value can be a variable or the property of an object. When doing so, you need to make a reference to the target element, and then use a native JavaScript or jQuery method to inject that value (e.g. [SOME_ELEMENT].innerHTML or $(SOME_ELEMENT).html() ). But if that value is injected into more than one element, your work grows exponentially. You now have to manage multiple DOM element references, write code that makes the injections, and also code that pays attention to the data you are working with, so that when that data changes, you can update the DOM element(s) that need to know about that update.
This scenario can quickly spiral into a frustrating mess of nested-callbacks, hard-coded DOM element references and data-handling functions.
One of the main features of Angular is “Directives.” Angular.js directives are extensions of HTML. They look / function much like HTML attributes; each directive is part of the element’s opening HTML tag, and requires a value, which is enclosed in double-quotes. The ng-model directive specifies the name of a piece of data, which one or more elements can be bound to.
Before we get started, we’ll need to let Angular.js know that we want it to manage our web page. We can do this by adding an ng-app directive to the markup that we want managed (or an element on the page that is a descendant of that markup, such as HTML or BODY).
Example # 1
1 |
<body ng-app> |
In Example # 1, we see the ng-app directive. This simply tells Angular to manage everything inside of the BODY tag. We could have placed this close to the elements that we actually want Angular so manage, but it is most simple, and not uncommon, to place it in the BODY tag.
Example # 2
1 2 |
<input type="text" ng-model="myData" /> <p class="content"><b>The Value of NG-MODEL is:</b><span class="val">{{myData}}</span></p> |
In Example # 2, we have a text box (an input that has a type attribute of “text”), and a paragraph. We have provided an ng-model attribute for the input element, and provided a value of “myData”. Behind the scenes, Angular has created an object that is a private variable of a function, given it a property named “myData”, and bound the value of it to this element. Anything that we type into this textbox will become the updated value of the “myData” property, and when that same “myData” property is changed elsewhere, this textbox will reflect that new value. This two-way data-binding is one of the most powerful features of Angular.js. You’ll also notice that in the HTML of Example # 2, there is a set of double-curly braces, with the text “myData” inside of it. This tells Angular that the value of myData should go inside of the double-curly braces, and be updated whenever the value of myData changes. If you click the link below, it will take you to the JsFiddle.net link for the fully working demo of Example # 1’s code.
HERE IS THE JS-FIDDLE.NET LINK FOR EXAMPLE # 2: http://jsfiddle.net/L69aq/
How to Demo: Type anything in the text box. You’ll see that whatever you type is updated in the element below the text box. Both the text-box and the element that displays the text are bound to the “myData” property of the same object, which has been created by Angular.
Example # 3
1 2 3 4 5 6 |
<select ng-model="myData"/> <option>Small</option> <option>Medium</option> <option>Large</option> <option>Super-Size</option> </select> |
In Example # 3, we have added a select element to the page. We have included an Angular ng-model directive and specified a value of “myData”. This means that the select element is bound to the exact same data as the two elements in Example # 2’s code.
If you click the link below, it will take you to the JsFiddle.net link for the fully working demo of Example # 3’s code.
HERE IS THE JS-FIDDLE.NET LINK FOR EXAMPLE # 3: http://jsfiddle.net/L69aq/1/
How to Demo: In addition to typing something in the text box, you can now select one of the options from the drop-down box. Now that SELECT element, the text-box, and the element that displays the text are bound to the “myData” property of the same object, which has been created by Angular.
Summary
In this article, we covered the bare-bones basics of data-binding in Angular.js. The key component here is the ng-model directive. We discussed how the ng-model directive creates a property of an object that is a private variable of a function, and bound to any element whose ng-model directive’s value is the same. We also demonstrated that when more than one element’s ng-model directive values are the same, there is a two-way data binding because when one element changes the value of the data, the rest of the elements bound to that data are updated, and vice versa.
This article merely scratches the surface of data-binding in Angular.js, but the hope is that it has provided a foundation upon which you can start to understand the incredibly powerful and elegant JavaScript library.
Helpful Links for Angular.js Data Binding Basics
http://docs.angularjs.org/guide/databinding
[…] Data-binding with Angular.js is surprisingly simple. To get started, you need only specify an ng-model directive. […]
[…] Part I of this series: “Getting Started With Angular.js: Data-Binding Basics With the ng-model Directive (Part I),” we covered the absolute basics of data-binding with Angular.js. In that article, we discussed […]